Multiple Flutter screens or views
Scenarios
#If you're integrating Flutter into an existing app, or gradually migrating an existing app to use Flutter, you might find yourself wanting to add multiple Flutter instances to the same project. In particular, this can be useful in the following scenarios:
- An application where the integrated Flutter screen is not a leaf node of the navigation graph, and the navigation stack might be a hybrid mixture of native -> Flutter -> native -> Flutter.
- A screen where multiple partial screen Flutter views might be integrated and visible at once.
The advantage of using multiple Flutter instances is that each instance is independent and maintains its own internal navigation stack, UI, and application states. This simplifies the overall application code's responsibility for state keeping and improves modularity. More details on the scenarios motivating the usage of multiple Flutters can be found at flutter.dev/go/multiple-flutters.
Flutter is optimized for this scenario, with a low incremental memory cost (~180kB) for adding additional Flutter instances. This fixed cost reduction allows the multiple Flutter instance pattern to be used more liberally in your add-to-app integration.
Components
#The primary API for adding multiple Flutter instances on both Android and iOS is based on a new FlutterEngineGroup
class (Android API, iOS API) to construct FlutterEngine
s, rather than the FlutterEngine
constructors used previously.
Whereas the FlutterEngine
API was direct and easier to consume, the FlutterEngine
spawned from the same FlutterEngineGroup
have the performance advantage of sharing many of the common, reusable resources such as the GPU context, font metrics, and isolate group snapshot, leading to a faster initial rendering latency and lower memory footprint.
FlutterEngine
s spawned fromFlutterEngineGroup
can be used to connect to UI classes likeFlutterActivity
orFlutterViewController
in the same way as normally constructed cachedFlutterEngine
s.The first
FlutterEngine
spawned from theFlutterEngineGroup
doesn't need to continue surviving in order for subsequentFlutterEngine
s to share resources as long as there's at least 1 livingFlutterEngine
at all times.Creating the very first
FlutterEngine
from aFlutterEngineGroup
has the same performance characteristics as constructing aFlutterEngine
using the constructors did previously.When all
FlutterEngine
s from aFlutterEngineGroup
are destroyed, the nextFlutterEngine
created has the same performance characteristics as the very first engine.The
FlutterEngineGroup
itself doesn't need to live beyond all of the spawned engines. Destroying theFlutterEngineGroup
doesn't affect existing spawnedFlutterEngine
s but does remove the ability to spawn additionalFlutterEngine
s that share resources with existing spawned engines.
Communication
#Communication between Flutter instances is handled using platform channels (or Pigeon) through the host platform. To see our roadmap on communication, or other planned work on enhancing multiple Flutter instances, check out Issue 72009.
Samples
#You can find a sample demonstrating how to use FlutterEngineGroup
on both Android and iOS on GitHub.
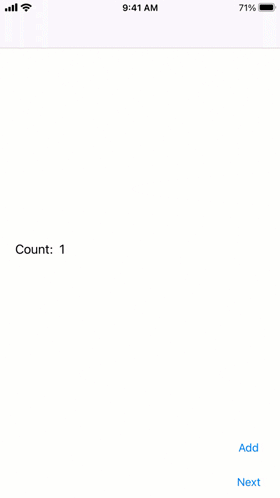
Unless stated otherwise, the documentation on this site reflects the latest stable version of Flutter. Page last updated on 2025-01-16. View source or report an issue.